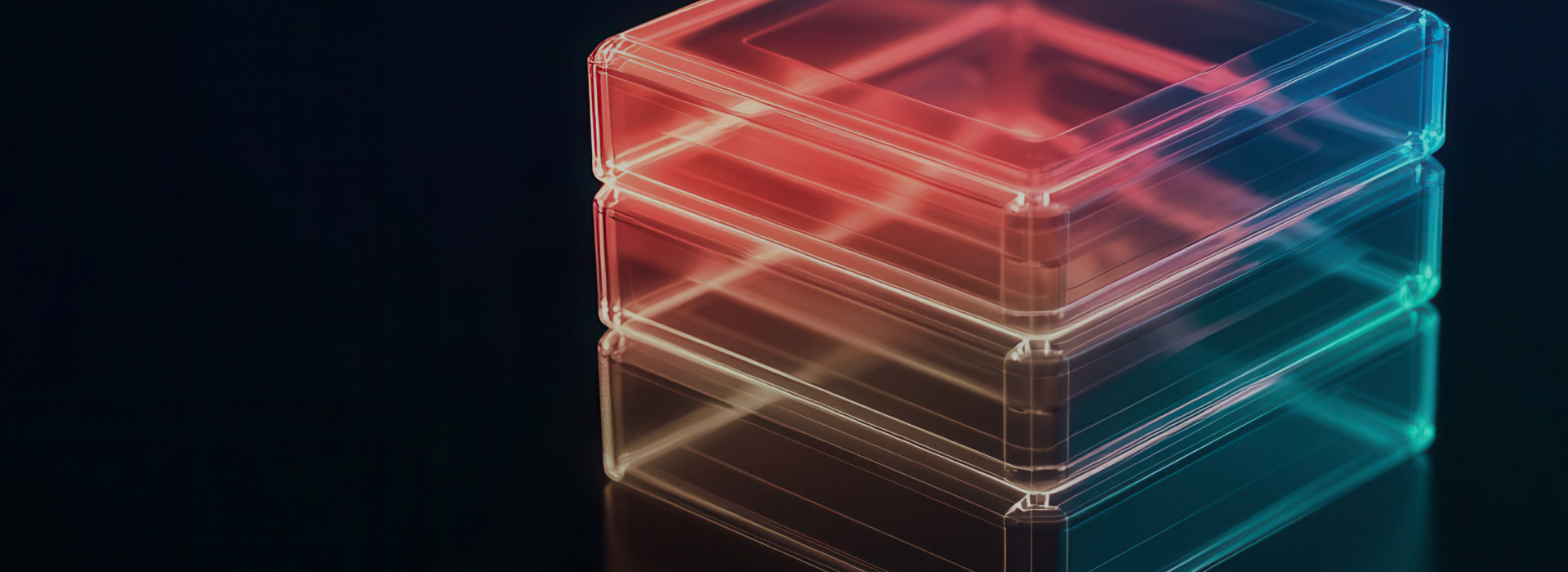
How to Develop a Virtual Disk Driver for Windows: A Practical Example
Learn how to develop a virtual disk driver for Windows that ensures secure data storage, seamless integration, and high system performance.
Meet Apriorit Team at Automotive Testing Expo Europe 2025
Halls 8 & 10, Messe Stuttgart, Germany | May 20-22
Learn how to develop a virtual disk driver for Windows that ensures secure data storage, seamless integration, and high system performance.
Looking for the best reverse engineering tools? Read our review of the top reverse engineering programs for different operating systems.
Explore the latest RSA Conference 2025 cybersecurity trends and learn how Apriorit can help organizations adapt to threats.
Learn how to reverse engineer software on Windows legally using proven techniques, essential tools, and insights from Apriorit experts.
Explore key benefits and use cases for AI in FinTech to make strategic decisions when enhancing your FinTech software solutions.
Enhance cybersecurity data visualization with advanced analytics, real-time threat insights, and intuitive visual models for faster threat detection.
Explore top FinTech cybersecurity risks and discover best practices to protect your app from threats, breaches, and compliance issues.
Discover how to build a secure AI chatbot with key risks, best practices, and strategies to protect data, users, and business systems.
We reveal business benefits of using MDM for logistics. Discover how centralized device management ensures data security and simplifies the IT routine...
Buy Now Pay Later (BNPL) solution development: market overview, must-have features, technology stack, and implementation challenges
Tell us about
your project
...And our team will:
Do not have any specific task for us in mind but our skills seem interesting? Get a quick Apriorit intro to better understand our team capabilities.