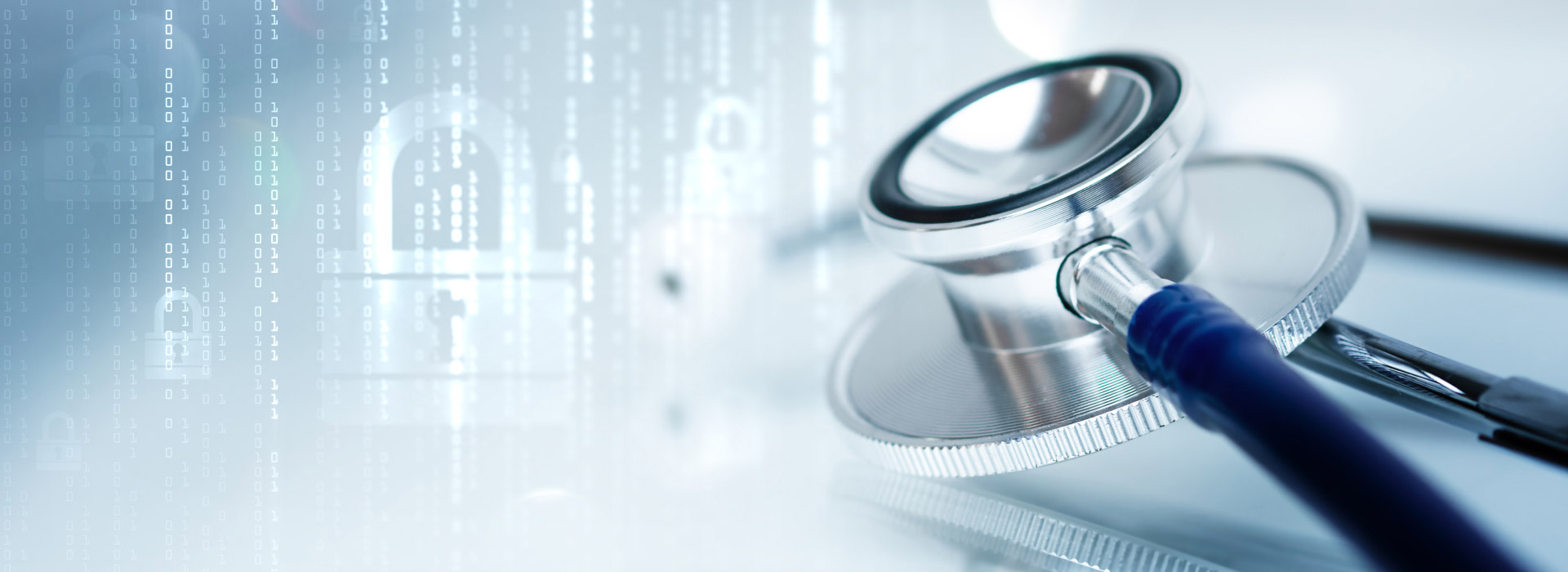
Cybersecurity Risks in Healthcare Software: Key Threats and How to Address Them
Discover key cybersecurity threats in healthcare, what puts patient data at risk, and best practices to protect systems from cyber attacks.
Discover key cybersecurity threats in healthcare, what puts patient data at risk, and best practices to protect systems from cyber attacks.
Choose the right open banking API management platform — AWS, Azure, or Apigee — to make your FinTech product secure, compliant, and scalable.
AI for demand forecasting: Explore business benefits, use cases, and Apriorit’s tips on how to create effective AI-based solutions.
Get your vehicle software closer to ISO 26262 compliance with key information on ensuring automotive functional safety.
Learn how to develop a virtual disk driver for Windows that ensures secure data storage, seamless integration, and high system performance.
Looking for the best reverse engineering tools? Read our review of the top reverse engineering programs for different operating systems.
Explore the latest RSA Conference 2025 cybersecurity trends and learn how Apriorit can help organizations adapt to threats.
Learn how to reverse engineer software on Windows legally using proven techniques, essential tools, and insights from Apriorit experts.
Explore key benefits and use cases for AI in FinTech to make strategic decisions when enhancing your FinTech software solutions.
Enhance cybersecurity data visualization with advanced analytics, real-time threat insights, and intuitive visual models for faster threat detection.
Tell us about
your project
...And our team will:
Do not have any specific task for us in mind but our skills seem interesting? Get a quick Apriorit intro to better understand our team capabilities.